Generating Hash Keys In Python
In a public key cryptography system, senders and receivers do not use the same key.Instead, the system defines a key pair, with one of the keys beingconfidential (private) and the other not (public).
Generating hash id’s using uuid3 and uuid5 in Python Python’s UUID class defines four functions and each generates different version of UUIDs. Let’s see how to generate UUID based on MD5 and SHA-1 hash using uuid3 and uuid5. Add this hash value to your outbound message in the format hash=yourhash (where yourhash is the hash you generated in step 3 above; N.B. For this example we were using an integration key of 3e9fed89-60e1-4ce5-ab6e-6b1eb2d4f977. You can test this with your hashing code to see if you get the same hash. Most pythonic way to generate a URL safe unique key or token is to use secrets module. Use secrets.tokenurlsafe it will return a secure random URL-safe text string. The secrets module uses synchronization methods to ensure that no two processes can obtain the same data at the same time.
Algorithm | Sender uses. | Receiver uses… |
---|---|---|
Encryption | Public key | Private key |
Signature | Private key | Public key |
- Generating Strong Password using Python Having a weak password is not good for a system which demands high confidentiality and security of user credentials. It turns out that people find it difficult making up a strong password which is strong enough to prevent unauthorized users from memorizing it.
- But this is a bad hash function because it means that all (key, value) pairs will be placed in a single list, and so each lookup will require searching this entire list. Thus a (very) desirable property of a hash function is that if two keys produce the same hash values, then the key objects are equivalent, that is.
Unlike keys meant for symmetric cipher algorithms (typically justrandom bit strings), keys for public key algorithms have very specificproperties. This module collects all methods to generate, validate,store and retrieve public keys.
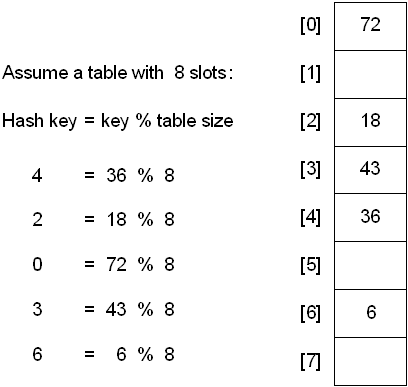
API principles¶
Asymmetric keys are represented by Python objects. Each object can be eithera private key or a public key (the method has_private()
can be usedto distinguish them).
A key object can be created in four ways:
Ssl private key generator. SSL.com’s public CSR and Key Generator is currently down for maintenance as part of our website’s redesign and update. We will be back soon with a new and updated version. In the mean time, we encourage our customers to learn about generating CSRs and keys in. Jul 09, 2019 Public Key Infrastructure (PKI) security is about using two unique keys: the Public Key is encrypted within your SSL Certificate, while the Private Key is generated on your server and kept secret. All the information sent from a browser to a website server is encrypted with the Public Key, and gets decrypted on the server side with the Private Key.
generate()
at the module level (e.g.Crypto.PublicKey.RSA.generate()
).The key is randomly created each time.import_key()
at the module level (e.g.Crypto.PublicKey.RSA.import_key()
).The key is loaded from memory.construct()
at the module level (e.g.Crypto.PublicKey.RSA.construct()
).The key will be built from a set of sub-components.publickey()
at the object level (e.g.Crypto.PublicKey.RSA.RsaKey.publickey()
).The key will be the public key matching the given object.
A key object can be serialized via its export_key()
method.
Generating Hash Keys In Python Pdf
Keys objects can be compared via the usual operators and !=
(note that the two halves of the same key,private and public, are considered as two different keys).