Generate List From Dictionary With Common Key Python
Python: How to create a list of all the keys in the Dictionary? Python: How to add / append key value pairs in dictionary using dict.update Python: How to get all keys with maximum value in a Dictionary; Python: Find duplicates in a list with frequency count & index positions; Python: How to check if a key exists in dictionary? Python dictionary method keys returns a list of all the available keys in the dictionary. Following is the syntax for keys method − dict.keys Parameters. Return Value. This method returns a list of all the available keys in the dictionary. The following example shows the usage of keys method. Given a list, write a Python program to convert the given list to dictionary such that all the odd elements have the key, and even number elements have the value. Since python dictionary is unordered, the output can be in any order. Automatically add key to Python dict. I want to automatically add keys to a Python dictionary if they don't exist already. Check if a given key already exists.
- Generate List From Dictionary With Common Key Python Free
- Python Generate Dictionary From List Of Keys
- Python Basic Tutorial
- Python Advanced Tutorial
- Python Useful Resources
- Selected Reading
Dictionary data type is used in python to store multiple values with keys. A new dictionary can be created by merging two or more dictionaries. Many ways exist in Python for merging dictionaries. How you can merge dictionaries are shown in this article by using various examples. Given a list, write a Python program to convert the given list to dictionary such that all the odd elements have the key, and even number elements have the value. Since python dictionary is unordered, the output can be in any order.
Each key is separated from its value by a colon (:), the items are separated by commas, and the whole thing is enclosed in curly braces. An empty dictionary without any items is written with just two curly braces, like this: {}.
Keys are unique within a dictionary while values may not be. The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples.
Accessing Values in Dictionary
To access dictionary elements, you can use the familiar square brackets along with the key to obtain its value. Following is a simple example −
When the above code is executed, it produces the following result −
If we attempt to access a data item with a key, which is not part of the dictionary, we get an error as follows −
When the above code is executed, it produces the following result −
Updating Dictionary
You can update a dictionary by adding a new entry or a key-value pair, modifying an existing entry, or deleting an existing entry as shown below in the simple example −
When the above code is executed, it produces the following result −
Delete Dictionary Elements
You can either remove individual dictionary elements or clear the entire contents of a dictionary. You can also delete entire dictionary in a single operation.
To explicitly remove an entire dictionary, just use the del statement. Following is a simple example −
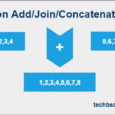
This produces the following result. Note that an exception is raised because after del dict dictionary does not exist any more −
Note − del() method is discussed in subsequent section.
Properties of Dictionary Keys
Dictionary values have no restrictions. They can be any arbitrary Python object, either standard objects or user-defined objects. However, same is not true for the keys.
There are two important points to remember about dictionary keys −
(a) More than one entry per key not allowed. Which means no duplicate key is allowed. When duplicate keys encountered during assignment, the last assignment wins. For example −
When the above code is executed, it produces the following result −
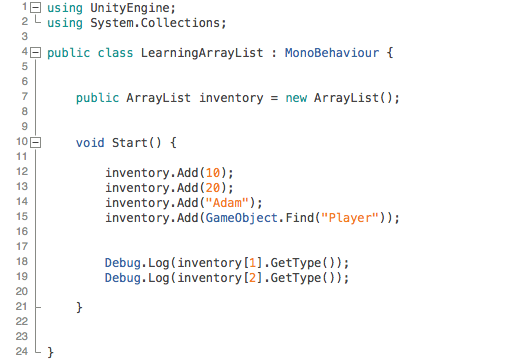
(b) Keys must be immutable. Which means you can use strings, numbers or tuples as dictionary keys but something like ['key'] is not allowed. Following is a simple example −
When the above code is executed, it produces the following result −
Built-in Dictionary Functions & Methods
Python includes the following dictionary functions − Dragon ball raging blast 2 key generator.
Sr.No. | Function with Description |
---|---|
1 | cmp(dict1, dict2) Compares elements of both dict. |
2 | len(dict) Gives the total length of the dictionary. This would be equal to the number of items in the dictionary. |
3 | str(dict) Produces a printable string representation of a dictionary |
4 | type(variable) Returns the type of the passed variable. If passed variable is dictionary, then it would return a dictionary type. |
Python includes following dictionary methods −
Sr.No. | Methods with Description |
---|---|
1 | dict.clear() Removes all elements of dictionary dict |
2 | dict.copy() Returns a shallow copy of dictionary dict |
3 | dict.fromkeys() Create a new dictionary with keys from seq and values set to value. |
4 | dict.get(key, default=None) For key key, returns value or default if key not in dictionary |
5 | dict.has_key(key) Returns true if key in dictionary dict, false otherwise |
6 | dict.items() Returns a list of dict's (key, value) tuple pairs |
7 | dict.keys() Returns list of dictionary dict's keys |
8 | dict.setdefault(key, default=None) Similar to get(), but will set dict[key]=default if key is not already in dict |
9 | dict.update(dict2) Adds dictionary dict2's key-values pairs to dict |
10 | dict.values() Returns list of dictionary dict's values |
Example-1: Merge two simple dictionaries
update() method is used in python to combine one dictionary with another dictionary. The following example shows the use of update() method. Here, two dictionaries are declared named stdDic1 and stdDic2. The values of stdDic1 will be added at the end of stdDic2. Next, for loop is used to print the keys and values of the merged dictionary.
stdDic1 ={'Jony Lever':'English','Meena Ali':'CSE','John Micheal':'LAW'}
# Define a dictionary of student list2
stdDic2 ={'John Abraham':'CSE','Mily Hossain':'BBA','Ella Binte Nazir':'EEE'}
# Merge the second dictionary with the first dictionary
stdDic2.update(stdDic1)
# Print the keys and values of the merged dictionary
for val in stdDic2:
print('nName:',val)
print('Department:',stdDic2[val])
Output:
Run the script. The following output will appear after running the script.
Generate List From Dictionary With Common Key Python Free
Example-2: Merge a simple dictionary and a list of multiple dictionaries
The following example shows how you can merge a dictionary with a list of multiple dictionaries. Here, a dictionary named isbn is declared to store the ISBN of the book as a key and book type as value. A list of dictionaries named book is declared to store book title and author name. zip() method is used to join the dictionaries or tuples and dict() method is used to create a dictionary. These methods are used in this script to create a new dictionary by merging isbn and book. Next, for loop is used to access the values of the merged dictionary.
isbn ={'67533344':'PHP','997544333':'Java','456688644':'VB.net'}
# Declare a list of multiple dictionary
book =[{'title': 'Murach PHP and MySQL','author': 'Joel Murach and Ray Harris'},
{'title': 'Java The Complete Reference','author': 'Herbert Schildt'},
{'title': 'Beginning VB.NET','author': 'Blair Richard, Matthew Reynolds, and
Thearon Willis'}]
# Create a new dictionary by merging a single and multiple dictionary
mrgDict =dict(zip(isbn, book))
# Print the keys and values of the merged dictionary
for isbn in mrgDict:
print('nISBN:',isbn)
print('Book Name:',mrgDict[isbn]['title'])
print('Author Name:',mrgDict[isbn]['author'])
Output:
Run the script. The following output will appear after running the script.
Example-3: Merge two dictionaries using custom function
Two dictionaries can be merged by using copy() and update() methods in python. Here, the original values of the dictionary will be unchanged. mergeDic() function is defined to copy the values of the first dictionary in a variable named merged and add the values of the second dictionary in merged. Next, the values of the merged dictionary is printed.
dict1 ={'name': 'Abir','age': 25,'gender': 'Male'}
dict2 ={'profession': 'Programmer','email': 'abir@gmail.com'}
'' Define a function to create a new dictionary merging both keys
and values, of dict1 and dict2''
def mergeDict(d1, d2):
merged = d1.copy()
merged.update(d2)
return merged
# Call the function to merge
mrgDict = mergeDict(dict1,dict2)
# Print the values of merged dictionary
for idval in mrgDict:
print(idval,':',mrgDict[idval])
Output:
Run the script. The following output will appear after running the script.
Example-4: Merging two dictionaries using (**) operator
Dictionaries can be merged without using a built-in or custom function by using a single expression. ‘**’operator is used in this example to merge two dictionaries. Here, two dictionary variables named dict1 and dict2 are declared, merged by using ‘**’ operator with the dictionary variables and stores the values into the variable, mrgDict.
dict1 ={'Moniter': 500,'Mouse': 100,'Keyboard': 250}
dict2 ={'HDD': 300,'Printer': 50,'Mouse':50}
# Merge dictionaries using '**' operator
mrgDict ={**dict2, **dict1}
# Print the values of merged dictionary
for val in mrgDict:
print(val,':',mrgDict[val])
Output:
Run the script. The following output will appear after running the script.
Python Generate Dictionary From List Of Keys
Example-5: Merging two dictionaries based on common keys
When two dictionaries contain the same key and if the value of the key is numeric then it may require to sum the values at the time of merging. This example shows how the numeric values of the same keys can be added when merging two dictionaries. Here, two dictionaries named store1 and store2 are declared. The keys and values of store1 are iterated through for loop and check which keys of store1 are equal to the keys of store2. If any key exists then the values of the key will be added.
store1 ={'Pen': 150,'Pencil': 250,'Note Book': 100}
store2 ={'Eraser': 80,'Pen': 50,'Sharpner': 30,'Pencil': 100}
# Merge the values of store2 with store1 with the common keys
for key in store1:
if key in store2:
store1[key]= store1[key] + store2[key]
else:
pass
# Print the keys and values of the merged dictionary
for val in store1:
print(val,':',store1[val])
Output:
Run the script. Here, two keys are common in the dictionaries. These are ‘Pen’ and ‘Pencil’ and the values of these keys are added.
Example-6: Merging all values of the dictionaries by counting common keys
In the previous example, the common values of two dictionaries are added based on a particular dictionary. This example shows how to merge the values of two dictionaries and add the values of common keys at the time of merging. Counter() method is used in the script to add the values of common keys.
fromcollectionsimport Counter
# Declare two dictionaries
store1 ={'Pen': 150,'Pencil': 250,'Note Book': 100}
store2 ={'Eraser': 80,'Pen': 50,'Sharpner': 30,'Pencil': 100}
# Merge the values of dictionaries based on common keys
mrgDic=Counter(store1)+Counter(store2)
# Print the keys and values of the merged dictionary
for val in mrgDic:
print(val,':',mrgDic[val])
Output:
Run the script. Here, one dictionary contains three elements and another dictionary contains four elements. Two keys are common in two dictionaries.
It was really hard to break the codes and access the main game components, but our team of professional coders have finally did it Video Tutorial. Nfs hot pursuit product key generator reviews. We worked on this CD Key Hack really hard, so in return we expect you to appreciate our work. Just in a few clicks you are able to generate serial keys.– Unlimited Keys (Don’t abuse the software please)– Slick looking new updated 2013 GUI (Optional settings)– Silent Load and functions– Working Keys for 32/64 bit systems– Virus Safe: ScreenshotWe are extremely happy that we can share it with you. All you have to do is download this tool and click on ”Generate Key” button and wait about 1 min.Need for Speed Hot Pursuit Key Generator (Key Hack) is finally available to download. hdwplayer id=37As you can see in our video it’s pretty easy to get some free Need for Speed Hot Pursuit 2010 CD Keys.
Conclusion:
You can merge two or more dictionaries based on your programming requirements. I hope, merging dictionaries will be an easy task for python users after practicing the above examples.